Python is a commonly utilized top-level, general-purpose programs language. The language uses numerous advantages to designers, making it a popular option for a vast array of applications consisting of web advancement, backend advancement, artificial intelligence applications, and all innovative software application innovation, and is chosen for both novices along with knowledgeable software application designers.
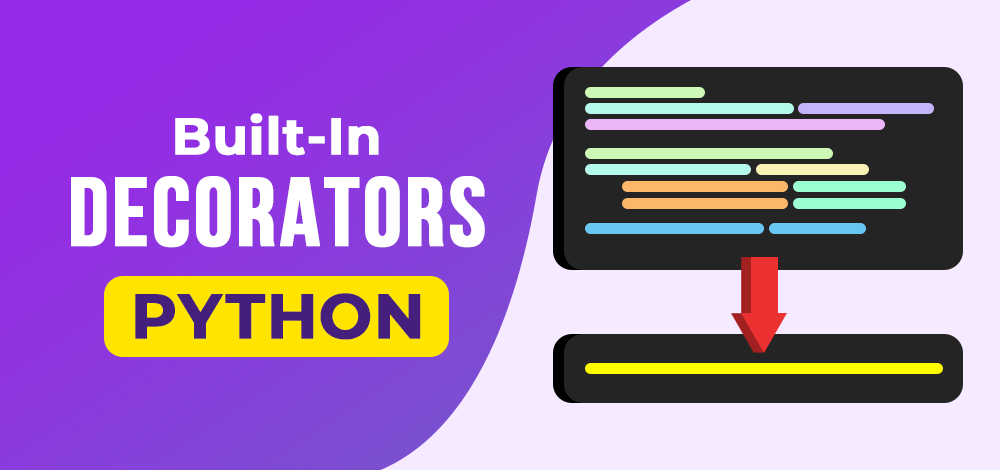
Python designers are important tools for composing tidy, flexible, multiple-use, and maintainable code, and as an outcome, are utilized thoroughly by software application designers Designers enable you to extend the habits of a function or class without altering its source code. They are likewise extensively utilized in numerous Python structures and libraries, such as Flask, Django, and SQLAlchemy In addition, there are numerous incredible integrated Python designers that make our lives a lot easier due to the fact that we can include complex performances to existing functions or classes with simply one line of code. Designers are typically utilized in Python for caching, logging, timing, authentication, permission, and recognition. With this being stated let’s continue the short article on the finest Python integrated designers to help you in having a a lot easier time finding out and constructing with Python.
Leading 10 Python Built-In Decorators That Optimize PythonCode Substantially
1. @classmethod
Class techniques are techniques that are bound to a specific class. These techniques are not connected to any circumstances of the class. The ‘ @classmethod’ is an integrated designer that is utilized to produce a class approach.
Example:
Python3
|
Output:
This is a Class approach . Worth of class variable 10
In the above execution, a class GFGClass and a class approach gfg_class_method are specified. The @classmethod designer is utilized to show that this is a class approach. The cls specification in the approach meaning describes the class itself, through which the class-level variables (example: class_val) and techniques can be accessed. When gfg_class_method() approach is called it logs the message, ” This is a Class approach” followed by the worth of the class_var
Likewise Check Out: @classmethod in Python
2. @abstractmethod
Abstract techniques are stated inside an Abstract class with no approach meaning. This approach is implied to be executed by the base class who carries out the moms and dad abstract class. The @abstractmethod designer offered by abc module is utilized to carry out abstract techniques.
Python3
|
Output:
100
In the above execution, The Forming class is specified as an abstract base class by acquiring from ABC and utilizing the @abstractmethod designer to state the location() approach as abstract. The Square class is a concrete subclass of Forming that carries out the location() approach to compute the location of a square. By acquiring from Forming, Square needs to carry out all of the abstract techniques stated in Forming. In this case, the Square class carries out location() by squaring the length of one side of the square.
Likewise, Read: @abstractmethod in Python
3. @staticmethod
Fixed techniques are merely energy techniques that are not bound to a class or a circumstances of the class. The ‘ @staticmethod‘ is an integrated designer that is utilized to produce a fixed approach.
Example:
Python3
|
Output:
This is a fixed approach
In the above execution, a class GFGClass and a fixed approach gfg_static_method are specified. The @staticmethod designer is utilized to show that this is a fixed approach. Unlike class techniques, fixed techniques do not have an unique very first specification that describes the class itself. Fixed techniques are frequently utilized for energy functions that do not depend upon the class or the circumstances of the class. When the gfg_static_method() approach is gotten in touch with the GFGClass class, the message “ This is a fixed approach” is logged to the console.
Likewise, Read: @staticmethod in Python
4. @atexit. register
The ‘ @atexit. register’ designer is utilized to call the function when the program is leaving. The performance is offered by the ‘ atexit’ module. This function can be utilized to carry out the clean-up jobs, prior to the program exits. Clean-ups are the jobs that have actually been performed in order to launch the resources such as opened files or database connections, that were being utilized throughout the program execution.
Example:
Python3
|
Output:
Hey There, Geeks! . Bye, Geeks!
In the above execution, a function gfg_exit_handler() is specified which utilizes the @atexit. register designer that registers it to be called when the program is leaving. When we run the program, the message “Hey There, Geeks!” is logged into the console. When finishes its execution either or by being cut off, the gfg_exit_handler() function is called and the message ” Bye, Geeks!” is logged to the console. The gfg_exit_handler() function can be beneficial for carrying out clean-up jobs or closing resources ( such as files or database connections) when a program is leaving.
Likewise, Read: @atexit. register in Python
5. @typing. last
The ‘ @final’ designer from the typing module is utilized to specify the last class or approach. Last classes can not be acquired and in a comparable method, last techniques can not be bypassed.
Example:
Python3
|
In the above execution, the @typing. last designer is utilized to show that a technique or class ought to not be bypassed or subclassed, respectively. The Base class has actually a done() approach marked as last utilizing @typing. last. The Kid is a subclass of Base that tries to bypass the done() approach, which raises a mistake when the code is performed. Likewise, the Geek class is marked as last utilizing @typing. last, showing that it must not be subclassed. The Other class efforts to subclass Leaf, which likewise raises a mistake when the code is performed.
Utilizing @typing. last can assist avoid unexpected modifications to crucial parts of your code and make it more robust by implementing restraints on how it can be utilized.
Likewise Check Out: @typing. last in Python
6. @enum. special
Enumeration or Enum is a set of special names that have a distinct worth. They work if we wish to specify a set of constants that have a particular significance. The ‘ @unique’ designer offered by enum module is utilized to guarantee that enumeration members are special.
Example:
Python3
|
In the above execution, an enum class ‘ Days’ is specified utilizing the class ‘ Enum‘. The class has 4 members, MONDAY, TUESDAY, WEDNESDAY, and THURSDAY, with worths of 1,2,3,2 respectively.
Nevertheless, due to the fact that TUESDAY and THURSDAY both have the worth 2, the @unique designer raises a ValueError with the message “ replicate worths discovered in << enum 'Days'>>: THURSDAY -> > TUESDAY” This mistake takes place due to the fact that the @unique designer makes sure that the worths of the enumeration members are special, and in this case, they are not.
Likewise, Read: @enum in Python
7. @property
Getters and Setters are utilized within the class to gain access to or upgrade the worth of the item variable within that class. The ‘ @property’ designer is utilized to specify getters and setters for class qualities.
Example:
Python3
|
Output:
Setter called . Getter Called . 10(* )In the above execution, the
Geek class has a personal associate ‘ _ name ‘. The program specifies the getter approach’ name ‘ which permits the name to be recovered utilizing the dot notation. The @name. setter designer is utilized to produce the setter approach for setting the’ _ name’ worth. The @property and @name. setter designers specify the” getter” and ” setter” techniques for class qualities, which can make it simpler to deal with class information. Likewise, Read:
@property in Python 8. @enum. confirm
Presented in Python 3.11, The
‘ @enum. confirm ‘ designer in Python is utilized to guarantee that the worths of the members of an enumeration follow a specific pattern or restraint. Some predefined restraints offered by the enum consist of, CONTINUES:
- The CONTINUES modules assist guarantee that there is no missing worth in between the greatest and most affordable worth. UNIQUE:
- The SPECIAL modules assist guarantee that each worth has just one name Example:
Python3
from
|
Days1 and Days2 are specified utilizing the Enum class from the enum module and the confirm() designer is used to each class. In the
Days1 class, the confirm() designer with the SPECIAL argument is utilized to guarantee that each member of the Days1 enumeration class has a distinct worth. Nevertheless, as TUESDAY and THURSDAY have the exact same worth, a ValueError is raised with the message: “ ValueError: aliases discovered in << enum 'Days'>>: THURSDAY -> > TUESDAY“. In the
Days2 class, the confirm() designer with the CONTINUOUS argument is utilized to guarantee that each member of the Days2 enumeration class forms a constant series beginning with the very first member. Nevertheless, considering that the worth of 3 is missing out on and 4 is straight utilized, a ValueError is raised with the message: “ ValueError: void enum ‘Days’: missing out on worths 3“. Likewise Check Out:
@enum in Python 9. @singledispatch
A function with the exact same name however various habits with regard to the kind of argument is a
generic function The ‘ @singledispatch’ designer in Python is utilized to produce a generic function This permits a private to produce an overloaded function that can deal with several kinds of criteria. Example:
Python3
from
|
Function Called with an integer
. Function Called with a list
.
Function Called with a string
. Function Call with single argument
In the above execution, a function called
geek_func() is specified utilizing the @singledispatch designer. The @singledispatch designer informs Python that this function ought to be dispatched based upon the kind of its very first argument. 4 extra executions of geek_func() are specified utilizing the @geek_func. register designer. Each execution is embellished with the kind of argument it deals with. When geek_func() is called with an argument of a specific type, the execution embellished with the matching type is called. When the function call does not match any of the signed up executions, it defaults to the preliminary execution Likewise, Read:
@singledispatch in Python 10. @lru_cache
This designer returns the like
lru_cache( maxsize= None) by developing a thin wrapper around a dictionary lookup for the function arguments. Since it never ever requires to eliminate old worths, this is smaller sized and faster than lru_cache() with a size limitation. Example:
Python3
from
|
55
.-- 0.0009987354278564453 seconds--
. 55
.
-- 0.0 seconds--
Likewise, Read:
@lru_cache in Python In the above execution of the Fibonacci series, we are utilizing memoization with the
lru_cache designer from the functools module. The Fibonacci series is a series of numbers in which each number is the amount of the 2 preceding ones, beginning with 0 and 1. The
fibonacci function is taking an integer argument n and returns the n-th number in the Fibonacci series and If n is less than 2, then the function returns n itself. Otherwise, it recursively calculates the amount of the ( n-1)- th and ( n-2)- th numbers in the series. Conclusion
Python is a flexible and widely-used programs language, making it an excellent option for designers to carry out code that is utilized for a range of functions. This short article highlights a few of the most popular and widely-used designers readily available in Python. Designers work abstractions for extending your code with extra performances such as caching, automated retry, rate restricting, logging, and more. These not just offer effective performance however likewise streamline the code. Utilizing designers can offer designers with effective tools for composing clear, succinct, and effective code in Python. Whether you are a novice or a skilled designer, utilizing these designers will offer an excellent beginning point for enhancing your code and leveraging the power of Python.
Frequently Asked Questions on Python Decorators
Q1: What is a designer in Python?
Response
: Python Designers
are extremely effective tools that enable developers to extend the habits of a function or class. Q2: How to produce a designer in Python?
Response
: A designer can be developed by specifying a function that takes another function as an argument and returns a brand-new function that extends or customizes the initial function's habits.
Q3: Can several designers be utilized for the exact same function?
Response
: Yes! It is possible to have several designers on the exact same function.